How to Serve Image Stored in a Server Using Spring Boot
-
Chinthaka Dinadasa
- 06 Oct, 2020
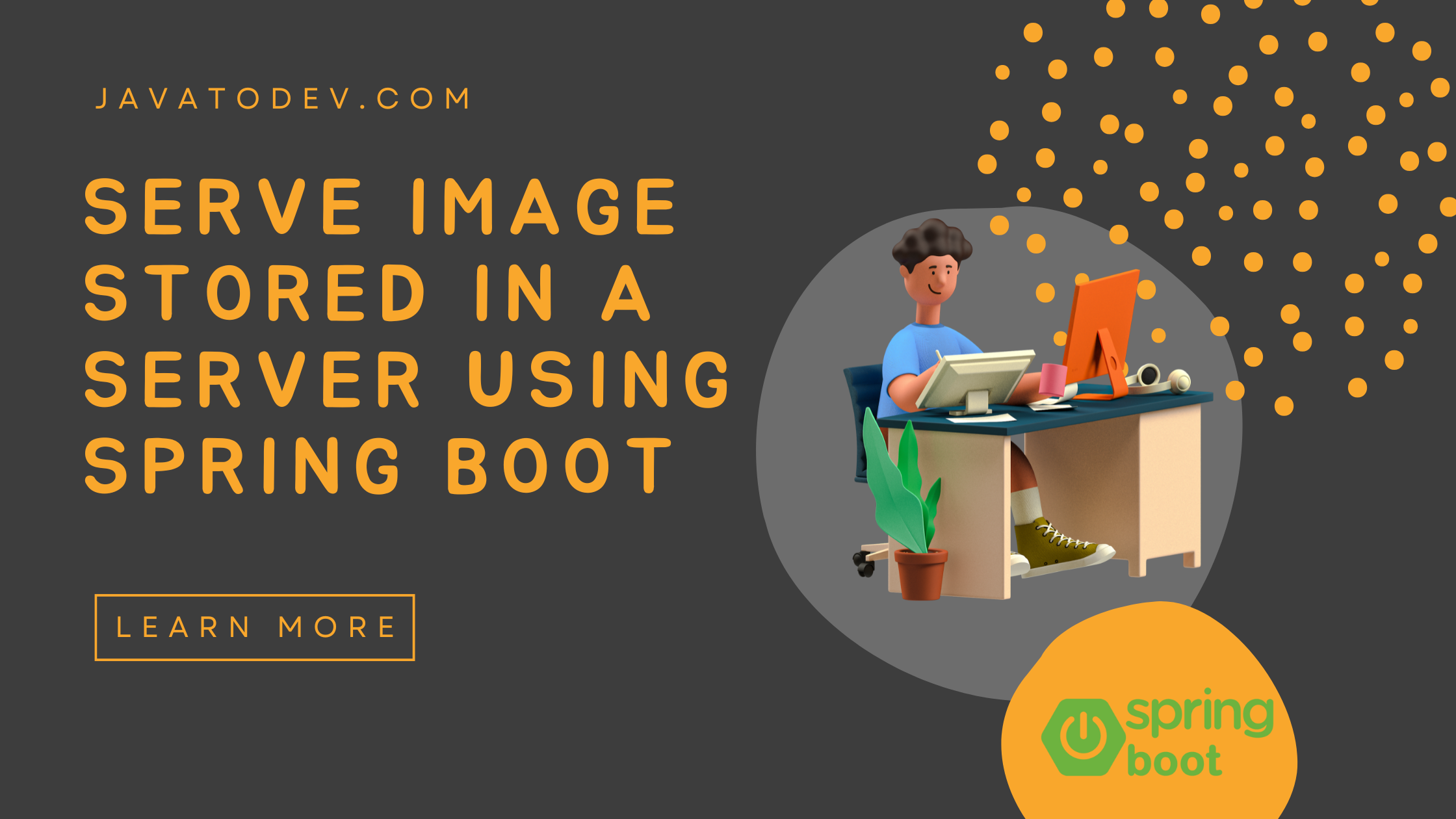
Let’s assume you need to create a direct link to serve an image file stored in a web server using Spring Boot. Here we are discussing how we can achieve that.
This tutorial is developed using Spring Boot, If you are really new to Spring Boot, Please follow our article on How to Create a Spring Boot Project.
Scenario: We have an image folder where we store multiple images inside a server. We need to read the image using a direct URL as below to use in the front end.
http://<host_name>:
eg: http://localhost:8080/images/pexels-ave-calvar-martinez-4852353.jpg
Adding GetMapping to Serve Image For Image Name
Here we need to introduce the GET endpoint to return Byte[] for the requested image setting return content type as MediaType.IMAGE_JPEG.
Additionally, we should use Apache Commons IO since we need to use FileUtils inside the Apache commons library.
We can add Apache Commons IO as a dependency by adding follow to build.gradle.
implementation 'commons-io:commons-io:2.8.0'
Java Implementation.
package com.javatodev.image.controller;
import com.javatodev.image.exception.ImageNotFoundException;
import org.apache.commons.io.FileUtils;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.io.File;
import java.io.IOException;
@RestController
@RequestMapping(value = "/images")
public class FileController {
//root path for image files
private String FILE_PATH_ROOT = "/opt/javatodev/images/";
@GetMapping("/{filename}")
public ResponseEntity<byte[]> getImage(@PathVariable("filename") String filename) {
byte[] image = new byte[0];
try {
image = FileUtils.readFileToByteArray(new File(FILE_PATH_ROOT+filename));
} catch (IOException e) {
e.printStackTrace();
}
return ResponseEntity.ok().contentType(MediaType.IMAGE_JPEG).body(image);
}
}
That’s all now we are ready to serve image content via a GET URL. Run the application and request images using a browser as follows.
eg: http://localhost:8080/images/pexels-ave-calvar-martinez-4852353.jpg
Handling 404 Error When Image Not Found
Here when there is no image found for a given path, It will send IOException, Hence we could handle that and send a proper 404 as a response.
To do that we can introduce a new Exception which returns 404 status.
package com.javatodev.image.controller;
import com.javatodev.image.exception.ImageNotFoundException;
import org.apache.commons.io.FileUtils;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.io.File;
import java.io.IOException;
@RestController
@RequestMapping(value = "/images")
public class FileController {
//root path for image files
private String FILE_PATH_ROOT = "/opt/javatodev/images/";
@GetMapping("/{filename}")
public ResponseEntity<byte[]> getImage(@PathVariable("filename") String filename) {
byte[] image = new byte[0];
try {
image = FileUtils.readFileToByteArray(new File(FILE_PATH_ROOT+filename));
} catch (IOException e) {
e.printStackTrace();
}
return ResponseEntity.ok().contentType(MediaType.IMAGE_JPEG).body(image);
}
}
And then throw it on IOException while FileUtils.readFileToByteArray.
try {
image = FileUtils.readFileToByteArray(new File(FILE_PATH_ROOT+filename));
} catch (IOException e) {
throw new ImageNotFoundException();
}
Conclusion
For this example, we’ve only used a JPEG formatted image set only. But this program could be changed to support multi-image types if you need by adding a few conditions.
In this article, we’ve discussed Serve Image Stored in a Server Using Spring Boot covering initial project setup, dependencies, API endpoint to return a byte[] for requested image file, and handling 404 error on conditions with Spring Boot.
You can find source codes for this tutorial from our Github.